Python program to print factorial of a given number
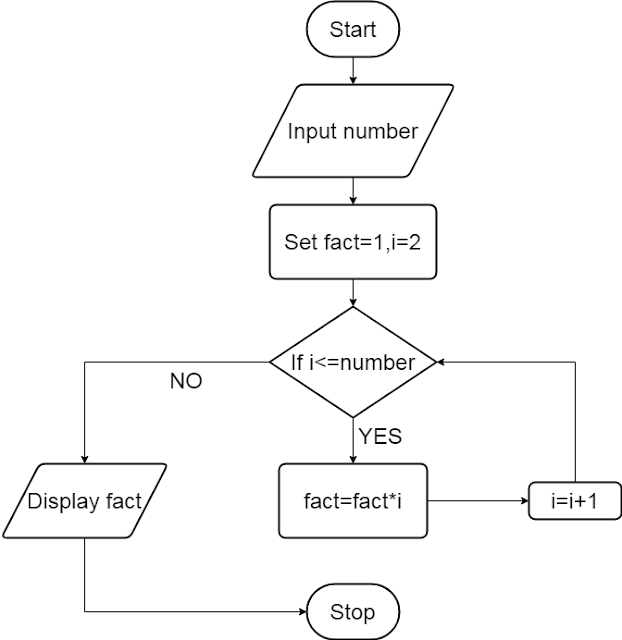
What are factorials? Factorial of an integer is product of that integer and all positive integers below it such that 4 factorial is equal to 4x3x2x1 = 24. Factorial is represented by "!" i.e. 4! means 4 factorial which is equal to 24. Algorithm :- Step 1- Start Step 2- Input a number Step 3- Declare variable fact and i Step 4- Initialize value fact←1 i←2 Step 5- if i<=number fact=fact*i i=i+1 repeat Step 5 else go to Step 6 Step 6- Display fact Step 7- Stop Flowchart :- Code :- number=int(input("Enter a number:")) fact=1 i=2 while i<=number: fact=fact*i i=i+1 print(fact)